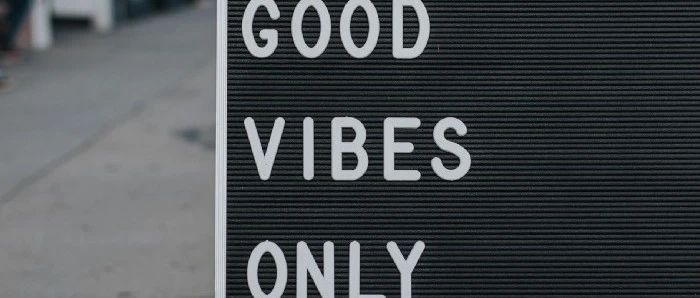
每个数据科学家都必须遵循的技巧
数据科学是一个涉及使用科学方法、过程、算法和系统从结构化和非结构化数据中提取知识和见解的领域。
数据科学家使用各种工具和技术,如机器学习、统计分析和可视化,来分析数据并从中获得见解。数据科学用于各种行业,如金融、医疗保健、营销和零售业,以帮助企业做出数据驱动的决策。
要在数据科学领域工作,你通常需要具备强大的编程、统计和数学技能,以及取决于你所从事行业的特定领域知识。
在这篇文章中,我们将介绍一些每个数据科学家为了获得更好的职业生涯必须了解的技巧。让我们开始吧!如果你想了解更多关于数据科学的相关内容,可以阅读以下这些文章:
每个数据科学家都应该养成的15个好习惯
将ChatGPT用于数据科学
你的第一份数据科学工作中要避免的错误
0经验?一样能成为一名成功的数据科学顾问!
1 编程语言
每个数据科学家都必须掌握Python和R等编程语言。这些语言通常用于数据科学中的数据操作、可视化和机器学习等任务。
下面是一些Python和R中的代码片段示例,演示了一些常见的数据操作和可视化任务:
Python:
# Import libraries
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
# Read in the dataset
df = pd.read_csv("data.csv")
# Fill missing values with the mean
df['column'] = df['column'].fillna(df['column'].mean())
# Create a histogram
plt.hist(df['column'])
plt.xlabel('Column')
plt.ylabel('Frequency')
plt.title('Histogram')
plt.show()
R:
# Install and load libraries
install.packages("ggplot2")
library(ggplot2)
# Read in the dataset
df <- read.csv("data.csv")
# Fill missing values with the mean
df$column[is.na(df$column)] <- mean(df$column, na.rm = TRUE)
# Create a histogram
ggplot(df, aes(x = column)) + geom_histogram() + labs(x = "Column", y = "Frequency", title = "Histogram")
2 了解数据操作和清理技术
数据科学家的大部分时间通常花在数据操作和清理任务上。作为一名数据科学家,你必须熟悉过滤、聚合和合并数据等技术。
Python语言中的Pandas库对于数据操作、清理和处理非常有用,并允许你执行各种操作,如数据过滤、聚合和合并。
Example:
import pandas as pd
# Read in the dataset
df = pd.read_csv("data.csv")
# Check for missing values
df.isnull().sum()
# Drop rows with missing values
df = df.dropna()
# Check for duplicates
df[df.duplicated()]
# Drop duplicates
df = df.drop_duplicates()
# Check for outliers
df.describe()
# Remove outliers
df = df[(df['column'] > lower_bound) & (df['column'] < upper_bound)]
# Save the cleaned dataset
df.to_csv("cleaned_data.csv", index=False)
这只是数据清理过程的一个示例,具体步骤将取决于数据集的特征和分析的目标。
3 学习数据可视化库和工具
能够有效地将数据可视化并进行交流是数据科学家的一项重要技能。作为一名数据科学家,您必须熟悉Matplotlib、Seaborn和Tableau等库和工具,这些库和工具可用于创建各种图表、图画和地图。
数据可视化是创建图表、图形和其他数据可视化表示以传达见解和发现的过程。下面是一个使用Python和matplotlib库的数据可视化示例:
import matplotlib.pyplot as plt
# Read in the dataset
df = pd.read_csv("data.csv")
# Create a histogram
plt.hist(df['x'])
plt.xlabel('X')
plt.ylabel('Frequency')
plt.title('Histogram')
plt.show()
# Create a scatter plot
plt.scatter(df['x'], df['y'])
plt.xlabel('X')
plt.ylabel('Y')
plt.title('Scatter Plot')
plt.show()
这只是使用matplotlib进行数据可视化的一个示例,还有许多其他类型的图表和图画可以使用此库或其他可视化库(如seaborn或plotly)创建。
4 机器学习算法
每个数据科学家都必须熟悉机器学习算法。作为一名数据科学家,你很可能会使用机器学习算法来分析数据并从中做出预测。
熟悉常见的算法,如线性回归、逻辑回归、决策树、随机森林和k-均值聚类,将有助于你成为一名数据科学家。
下面是一个使用Python和scikit-learn库的机器学习算法示例:
import numpy as np
from sklearn.linear_model import LinearRegression
# Read in the dataset
X = np.array([[1], [2], [3]])
y = np.array([4, 5, 6])
# Create the model
model = LinearRegression()
# Fit the model to the data
model.fit(X, y)
# Make predictions
predictions = model.predict([[4], [5], [6]])
print(predictions)
这个例子展示了如何使用线性回归,这是一种机器学习算法,用于根据一个或多个输入特征来预测一个连续值。
还有许多其他类型的机器学习算法,包括分类算法、聚类算法和深度学习算法,它们可以用于不同类型的任务和数据集。
5 使用数据集练习
练习使用大型数据集。数据科学家经常处理难以分析和操作的大型数据集,要熟悉处理大型数据集的技术,如分布式计算和流式数据处理。
要成为一名熟练的数据科学家,实际数据工作经验是必不可少的,因此要寻找机会参与涉及分析和解释真实数据的项目或练习。
有许多库和资源可用于在Python中访问数据集。下面是一些如何在Python中加载和使用数据集的示例:
# Load a dataset from a file
import pandas as pd
df = pd.read_csv("data.csv")
# Load a dataset from the web
import requests
url = "https://raw.githubusercontent.com/datasets/covid-19/master/data/countries-aggregated.csv"
df = pd.read_csv(url)
# Load a dataset from a database
import sqlite3
conn = sqlite3.connect("database.db")
df = pd.read_sql_query("SELECT * FROM table", conn)
# Access and manipulate the data
print(df.head()) # print the first few rows
print(df.describe()) # summarize the data
df['column'] # access a specific column
df[['column1', 'column2']] # access multiple columns
df.groupby('column').mean() # group the data and compute the mean
这些只是如何在Python中使用数据集的几个示例,还有许多其他函数和技术可用于访问、操作和分析数据。
6 学习SOL
结构化查询语言(SQL)是一种广泛用于管理和查询数据库的编程语言。作为一名数据科学家,为了从数据库中提取和分析数据,熟练使用SQL非常重要,这是许多数据科学项目的重要组成部分。
作为数据科学家,你需要熟悉这种查询语言的基本语法和命令。这包括用于选择、筛选和聚合数据以及用于创建和操作表和数据库的命令。
下面是一些基本SQL命令的示例:
-- Create a table
CREATE TABLE users (
id INTEGER PRIMARY KEY,
name TEXT,
email TEXT
);
-- Insert a row into the table
INSERT INTO users (name, email)
VALUES ('Alice', 'alice@example.com');
-- Select data from the table
SELECT * FROM users;
SQL是一种用于处理结构化数据的强大语言,许多数据库都使用它,包括MySQL、PostgreSQL和SQLite。
7 了解云计算
云计算在数据科学领域越来越受欢迎,因为它允许数据科学家按需处理大量的数据和计算资源。
AWS、Azure和GCP等云计算平台为数据科学任务提供了各种工具和服务,包括存储、计算和机器学习。熟悉这些平台对你的数据科学职业生涯很有帮助。
下面是一个数据科学家如何在Python中使用云计算和机器学习的例子,使用Amazon SageMaker库:
import boto3
import sagemaker
# Connect to the AWS account
boto3.setup_default_session(region_name='us-east-1')
# Create a Sagemaker client
client = boto3.client('sagemaker')
# Specify the input and output locations in S3
input_data = sagemaker.s3_input(s3_data='s3://my-bucket/data/train', content_type='csv')
output_data = sagemaker.s3_output(s3_data='s3://my-bucket/data/output')
# Create a model
model = sagemaker.estimator.Estimator(
image_name='image:1.0',
role='SageMakerRole',
train_instance_count=1,
train_instance_type='ml.m4.xlarge',
train_volume_size=30,
train_max_run=360000,
input_mode='File',
output_path=output_data,
sagemaker_session=sagemaker.Session())
# Train the model
model.fit({'train': input_data})
# Deploy the model
model.deploy(initial_instance_count=1, instance_type='ml.t2.medium')
# Invoke the model to make predictions
client.invoke_endpoint(EndpointName='endpoint-name', ContentType='text/csv', Body=input_data)
这个例子展示了如何使用Amazon SageMaker在AWS上训练和部署机器学习模型。
8 紧跟时代步伐
数据科学领域在不断发展,因此紧跟最新技术和最佳实践非常重要。这可能涉及阅读技术博客、参加会议或参加在线课程。
结论
这就是就是本文的全部内容。在本文中,我们讨论了每个数据科学家或想从事数据科学领域工作的人必须遵循的一些技巧。
感谢阅读!你还可以订阅我们的YouTube频道,观看大量大数据行业相关公开课:https://www.youtube.com/channel/UCa8NLpvi70mHVsW4J_x9OeQ;在LinkedIn上关注我们,扩展你的人际网络!https://www.linkedin.com/company/dataapplab/
原文作者:Pralabh Saxena
翻译作者:马薏菲
美工编辑:过儿
校对审稿:Chuang
原文链接:https://levelup.gitconnected.com/tips-that-every-data-scientist-must-follow-c5f5d31e20f2